1. Write a program that will accept the number of hours an employee worked, along with his/her hourly pay rate. Calculate and display their gross pay (hours * rate). An example is given below.
Sample Run of the Program:
Enter hours worked: 5.5
Enter hourly rate: 8.75
Your gross pay is: 48.125
Use the above as test data for your program.
|
2. Write a program that will accept a cost and a tax rate. It will then calculate tax and total, then display a “receipt” of the transaction. An example is given below:
Sample Run of the Program:
Enter the price: 19.99
Enter the tax rate: 0.075
Price: 19.99
Tax: 1.49925
Total: 21.48925
|
3. Write a program that will accept a student's name, their number of points on a math quiz and the number of points that the quiz is worth. Calculate and display the percentage of points earned on the quiz. An example is given below.
Sample Run of the Program:
Enter your name: Karel
Enter your quiz score: 55
Enter the quiz value: 80
Karel, your quiz percentage is: 68.75%
Use the above as test data for your program. |
4. Write a program that will determine the weight on the moon for two people. The program must ask for the names of the people and their weights on Earth. Moon weight = 0.17 x Earth weight. An example is given below:
Sample Run of the Program:
Enter name of person #1: Willy
Enter weight of person #1: 147
Enter name of person #2: Andy
Enter weight of person #2: 118
Willy weighs 24.99 pounds on the Moon
Andy weighs 20.06 pounds on the Moon |
5. Write a program that will accept two integers, and then outputs their sum, difference, product and quotient. Note that the symbol in Java for multiplication is the asterisk (*) and for division it is the forward slash (/). An example is given below:
Sample Run of the Program:
Enter an integer: 5
Enter another integer: 2
5 + 2 = 7
5 – 2 = 3
5 * 2 = 10
5 / 2 = 2.5
|
6. Write a program that will accept a number of yards feet and inches, then determine and print the number of total inches. Recall that there are 36 inches in a yard and 12 inches in a foot. An example is given below.
Sample Run of the Program:
Enter the yards: 3
Enter the feet: 5
Enter the inches: 2
Total Inches: 170
|
7. Now write a program that does the opposite of program #4. This time, ask for the total inches, then calculate and display the number of yards, feet and inches. This requires the use of the modulus (%) operator. An example is given below.
Sample Run of the Program:
Enter the Total Inches: 270
Yards: 7
Feet: 1
Inches: 6
|
8. Write a program that will calculate the cost of buying a given number of eggs, as input by the user. The price of the eggs is $1.00 per dozen and $0.10 each for any extra eggs beyond an even dozen. This also requires the use of the modulus operator. An example is given below.
Sample Run of the Program:
Enter number of eggs: 125
Purchase: 10 dozen and 5 extra eggs
Your bill comes to $10.50
|
9. Write a program that will accept an amount of change less than a dollar. It will then determine and output the best change for that amount (in other words, using the fewest coins). An example is given below:
Sample Run of the Program:
Enter the change amount: 64
Quarters: 2
Dimes: 1
Nickels: 0
Pennies: 4
|
10. No Ka Oi Vacation Properties rents vacation condominiums on the island of Maui with the following rate plan: $1500 for a 30-day rental (one month), $400 for a weekly rental, $100 per day for anything under a week. Write a program that will accept a number of days that a customer wishes to rent. The program will then determine and display a cost breakdown for the vacation (see below).
Sample Run of the Program:
Enter the number of days: 45
Months: 1
Weeks: 2
Days: 1
Total Cost = $2400
|
11. Write a program that will accept information for the purchase of some item (What is it called? How much does it cost? and How many do you want?). A total cost should be determined and a report should be displayed as shown in the example below. You will need to use the EasyFormat class to take care of decimal places and to make sure that the output lines up as is shown in the examples below.
Sample Run of the Program:
Item Name: thing
Item Cost: 11.80
Number of Items: 12
ITEM COST QTY TOTAL
thing 11.80 12 141.60 |
12. Write a program that will accept the radius of a circle, then determine and display the diameter, circumference and area of the circle. Recall the following formulas:
diameter = 2 * radius
circumference = 2 * radius * PI
area = PI * radius * radius
Note: Use a constant value for PI = 3.1416. Also, round displayed results to 1 decimal place.
Sample Run of the Program:
Enter the Radius: 4.5
Radius Diam Circ Area
4.5 9.0 28.3 63.6
|
13. Write a program that will calculate your percentage of points accumulated for a class. The input will include homework and test points accumulated as well as the number of points possible for each category. Output the percentages to 2 decimal places.
Sample Run of the Program:
Enter number of HW points possible: 200
Enter number of HW points accumulated: 125
Enter number of TEST points possible: 400
Enter number of TEST points accumulated: 306
Total Points: 431 out of 600
Percentages:
HW TESTS OVERALL
62.50 76.50 71.83
|
14. In a scientific experiment, the percent error is calculated as
Write a program to enter an actual and an expected value for a temperature in degrees Fahrenheit, and then determine the percent error. Note that you will need to multiply the above formula by 100 so that the answer is in percent form. Display the result to two decimal places.
Sample run of the program:
Actual = 30.8
Expected = 32.0
Percent Error = 3.75%
|
15. A certain bacteria grows according to the model

where I is the initial population, t is time in days, P is the population at time t. Write a program which will allow you to input the initial population of bacteria and a number of days. The program will then output the current population at the end of that number of days, rounded to two decimal places.
Sample run of the program:
Initial population: 50
Number of days: 5
After 5 days, the population grows from 50 to 268.91
|
16. Write a program that will ask for the hypotenuse and one leg of a right triangle. The program will then find the other leg. Recall again that Leg1 squared + Leg2 squared = Hyp squared. You will need to use a variation of this equation:
Sample Run of the Program:
Enter the hypotenuse: 10
Enter Leg1: 5
Leg2 = 8.7 |
17. The surface area (S) and volume (V) of a sphere are found as follows
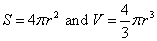
where r = radius and pi = 3.14. Ask the user for the radius of the sphere, then calculate and display the results (rounded to two decimal places, as shown). Hint: in the volume formula, express 4/3 as 4.0/3.0 to ensure correct decimal results.
Sample run of the program:
Enter the radius of the sphere: 4
Surface Area = 200.96 square units
Volume = 267.95 cubic units
|
18. The distance between two points (x1, y1) and (x2, y2) can be found using the equation

Ask the user for the coordinates of the two points, then calculate and display the result, rounded to one decimal place.
Sample Run of the Program:
Enter X1: 2
Enter y1: 3
Enter X2: 5
Enter y2: 2
The distance is 3.2 |